[2022年最新版] Laravel の開発に欠かせない Faker のチートシートを作ってみた
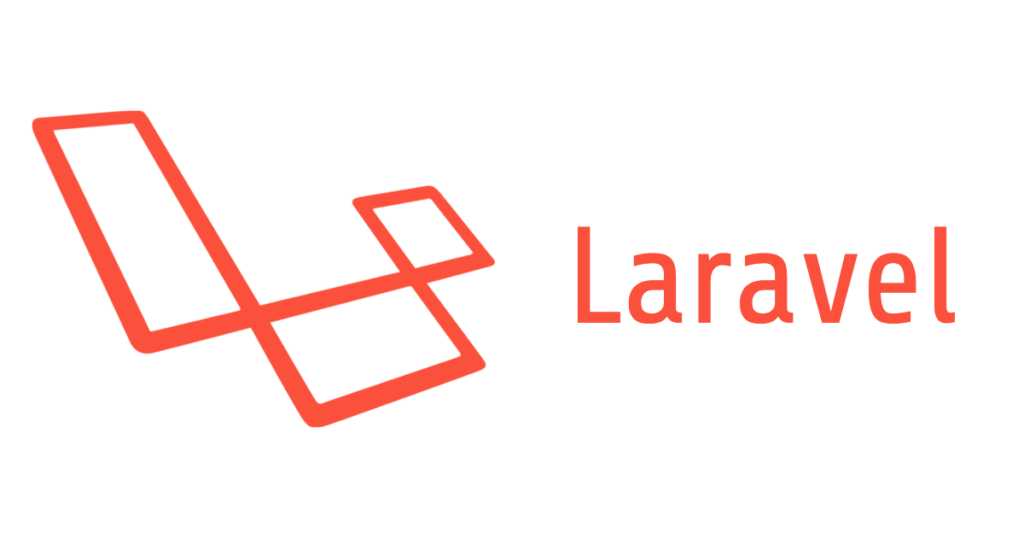
Laravel でモデルデータの作成に欠かせない Faker
ですが、最新バージョンのメソッド一覧がネットでなかなか見つからないので、スクリプトを書いて書き出しました。書き出し用のスクリプトは GitHub Gist に公開しています。
目次
- 1 Provider/Address.php
- 2 Provider/Barcode.php
- 3 Provider/Base.php
- 4 Provider/Biased.php
- 5 Provider/Color.php
- 6 Provider/Company.php
- 7 Provider/DateTime.php
- 8 Provider/File.php
- 9 Provider/HtmlLorem.php
- 10 Provider/Image.php
- 11 Provider/Internet.php
- 12 Provider/Lorem.php
- 13 Provider/Medical.php
- 14 Provider/Miscellaneous.php
- 15 Provider/Payment.php
- 16 Provider/Person.php
- 17 Provider/PhoneNumber.php
- 18 Provider/Text.php
- 19 Provider/UserAgent.php
- 20 Provider/Uuid.php
- 21 Provider/ja_JP/Address.php
- 22 Provider/ja_JP/Company.php
- 23 Provider/ja_JP/Internet.php
- 24 Provider/ja_JP/Person.php
Provider/Address.php
Method | Type | Example | Description |
---|---|---|---|
citySuffix() |
string |
'town' |
|
streetSuffix() |
string |
'Avenue' |
|
buildingNumber() |
string |
'791' |
|
city() |
string |
'Sashabury' |
|
streetName() |
string |
'Crist Parks' |
|
streetAddress() |
string |
'791 Crist Parks' |
|
postcode() |
string |
86039-9874 |
|
address() |
string |
'791 Crist Parks, Sashabury, IL 86039-9874' |
|
country() |
string |
'Japan' |
|
latitude($min = -90, $max = 90) |
float |
'77.147489' |
|
longitude($min = -180, $max = 180) |
float |
'86.211205' |
|
localCoordinates() |
float[] |
array('77.147489', '86.211205') |
Provider/Barcode.php
Method | Type | Example | Description |
---|---|---|---|
ean13() |
string |
'4006381333931' |
|
ean8() |
string |
'73513537' |
|
isbn10() |
string |
'4881416324' |
|
isbn13() |
string |
'9790404436093' |
Provider/Base.php
Method | Type | Example | Description |
---|---|---|---|
randomDigit() |
int |
Returns a random number between 0 and 9 | |
randomDigitNotNull() |
int |
Returns a random number between 1 and 9 | |
randomDigitNot($except) |
int |
Generates a random digit, which cannot be $except | |
randomNumber($nbDigits = null, $strict = false) |
int |
79907610 |
Returns a random integer with 0 to $nbDigits digits. |
randomFloat($nbMaxDecimals = null, $min = 0, $max = null) |
float |
48.8932 |
Return a random float number |
numberBetween($int1 = 0, $int2 = 2147483647) |
int |
79907610 |
Returns a random number between $int1 and $int2 (any order) |
passthrough($value) |
Returns the passed value | ||
randomLetter() |
string |
Returns a random letter from a to z | |
randomAscii() |
string |
Returns a random ASCII character (excluding accents and special chars) | |
randomElements($array = ['a', 'b', 'c'], $count = 1, $allowDuplicates = false) |
array New array with $count elements from $array |
Returns randomly ordered subsequence of $count elements from a provided array | |
randomElement($array = ['a', 'b', 'c']) |
Returns a random element from a passed array | ||
randomKey($array = []) |
int|string|null |
Returns a random key from a passed associative array | |
shuffle($arg = '') |
array|string The shuffled set |
$faker->shuffle('hello, world'); // 'rlo,h eold!lw' |
Returns a shuffled version of the argument. |
shuffleArray($array = []) |
array The shuffled set |
$faker->shuffleArray([1, 2, 3]); // [2, 1, 3] |
Returns a shuffled version of the array. |
shuffleString($string = '', $encoding = 'UTF-8') |
string The shuffled set |
$faker->shuffleString('hello, world'); // 'rlo,h eold!lw' |
Returns a shuffled version of the string. |
numerify($string = '###') |
string |
||
lexify($string = '????') |
string |
||
bothify($string = '## ??') |
string |
||
asciify($string = '****') |
string |
$faker->asciify(''********'); // "s5'G!uC3" |
|
regexify($regex = '') |
string |
$faker->regexify('[A-Z0-9._%+-]+@[A-Z0-9.-]+.[A-Z]{2,4}'); // sm0@y8k96a.ej |
|
toLower($string = '') |
string |
||
toUpper($string = '') |
string |
||
optional($weight = 0.5, $default = null) |
mixed|null |
||
unique($reset = false, $maxRetries = 10000) |
UniqueGenerator A proxy class returning only non-existing values |
||
valid($validator = null, $maxRetries = 10000) |
ValidGenerator A proxy class returning only valid values |
Provider/Biased.php
Method | Type | Example | Description |
---|---|---|---|
biasedNumberBetween($min = 0, $max = 100, $function = 'sqrt') |
int An integer between $min and $max. |
Returns a biased integer between $min and $max (both inclusive). |
Provider/Color.php
Method | Type | Example | Description |
---|---|---|---|
hexColor() |
string |
'#fa3cc2' |
|
safeHexColor() |
string |
'#ff0044' |
|
rgbColorAsArray() |
array |
'array(0,255,122)' |
|
rgbColor() |
string |
'0,255,122' |
|
rgbCssColor() |
string |
'rgb(0,255,122)' |
|
rgbaCssColor() |
string |
'rgba(0,255,122,0.8)' |
|
safeColorName() |
string |
'blue' |
|
colorName() |
string |
'NavajoWhite' |
|
hslColor() |
string |
'340,50,20' |
|
hslColorAsArray() |
array |
array(340, 50, 20) |
Provider/Company.php
Method | Type | Example | Description |
---|---|---|---|
company() |
string |
'Acme Ltd' |
|
companySuffix() |
string |
'Ltd' |
|
jobTitle() |
string |
'Job' |
Provider/DateTime.php
Method | Type | Example | Description |
---|---|---|---|
unixTime($max = 'now') |
int |
1061306726 |
|
dateTime($max = 'now', $timezone = null) |
DateTime |
DateTime('2005-08-16 20:39:21') |
|
dateTimeAD($max = 'now', $timezone = null) |
DateTime |
DateTime('1265-03-22 21:15:52') |
|
iso8601($max = 'now') |
string |
'2003-10-21T16:05:52+0000' |
|
date($format = 'Y-m-d', $max = 'now') |
string |
'2008-11-27' |
|
time($format = 'H:i:s', $max = 'now') |
string |
'15:02:34' |
|
dateTimeBetween($startDate = '-30 years', $endDate = 'now', $timezone = null) |
DateTime |
DateTime('1999-02-02 11:42:52') |
|
dateTimeInInterval($date = '-30 years', $interval = '+5 days', $timezone = null) |
DateTime |
dateTimeInInterval('1999-02-02 11:42:52', '+ 5 days') |
|
dateTimeThisCentury($max = 'now', $timezone = null) |
DateTime |
DateTime('1964-04-04 11:02:02') |
|
dateTimeThisDecade($max = 'now', $timezone = null) |
DateTime |
DateTime('2010-03-10 05:18:58') |
|
dateTimeThisYear($max = 'now', $timezone = null) |
DateTime |
DateTime('2011-09-19 09:24:37') |
|
dateTimeThisMonth($max = 'now', $timezone = null) |
DateTime |
DateTime('2011-10-05 12:51:46') |
|
amPm($max = 'now') |
string |
'am' |
|
dayOfMonth($max = 'now') |
string |
'22' |
|
dayOfWeek($max = 'now') |
string |
'Tuesday' |
|
month($max = 'now') |
string |
'7' |
|
monthName($max = 'now') |
string |
'September' |
|
year($max = 'now') |
string |
'1673' |
|
century() |
string |
'XVII' |
|
timezone() |
string |
'Europe/Paris' |
|
setDefaultTimezone($timezone = null) |
DateTime |
||
getDefaultTimezone() |
string|null |
Provider/File.php
Method | Type | Example | Description |
---|---|---|---|
mimeType() |
string |
'video/avi' |
|
fileExtension() |
string |
avi |
|
file($sourceDirectory = '/tmp', $targetDirectory = '/tmp', $fullPath = true) |
string |
Provider/HtmlLorem.php
Method | Type | Example | Description |
---|---|---|---|
randomHtml($maxDepth = 4, $maxWidth = 4) |
string |
Provider/Image.php
Method | Type | Example | Description |
---|---|---|---|
imageUrl($width = 640, $height = 480, $category = null, $randomize = true, $word = null, $gray = false) |
string |
'http://via.placeholder.com/640x480.png/CCCCCC?text=well+hi+there' |
Generate the URL that will return a random image |
image($dir = null, $width = 640, $height = 480, $category = null, $fullPath = true, $randomize = true, $word = null, $gray = false) |
bool|string |
'/path/to/dir/13b73edae8443990be1aa8f1a483bc27.png' |
Provider/Internet.php
Method | Type | Example | Description |
---|---|---|---|
email() |
string |
'jdoe@acme.biz' |
|
freeEmail() |
string |
'jdoe@gmail.com' |
|
companyEmail() |
string |
'jdoe@dawson.com' |
|
freeEmailDomain() |
string |
'gmail.com' |
|
userName() |
string |
'jdoe' |
|
password($minLength = 6, $maxLength = 20) |
string |
'fY4èHdZv68' |
|
domainName() |
string |
'tiramisu.com' |
|
domainWord() |
string |
'faber' |
|
tld() |
string |
'com' |
|
url() |
string |
'http://www.runolfsdottir.com/' |
|
slug($nbWords = 6, $variableNbWords = true) |
string |
'aut-repellat-commodi-vel-itaque-nihil-id-saepe-nostrum' |
|
ipv4() |
string |
'237.149.115.38' |
|
ipv6() |
string |
'35cd:186d:3e23:2986:ef9f:5b41:42a4:e6f1' |
|
localIpv4() |
string |
'10.1.1.17' |
|
macAddress() |
string |
'32:F1:39:2F:D6:18' |
Provider/Lorem.php
Method | Type | Example | Description |
---|---|---|---|
word() |
string |
'Lorem' |
|
words($nb = 3, $asText = false) |
array|string |
array('Lorem', 'ipsum', 'dolor') |
Generate an array of random words |
sentence($nbWords = 6, $variableNbWords = true) |
string |
'Lorem ipsum dolor sit amet.' |
Generate a random sentence |
sentences($nb = 3, $asText = false) |
array|string |
array('Lorem ipsum dolor sit amet.', 'Consectetur adipisicing eli.') |
Generate an array of sentences |
paragraph($nbSentences = 3, $variableNbSentences = true) |
string |
'Sapiente sunt omnis. Ut pariatur ad autem ducimus et. Voluptas rem voluptas sint modi dolorem amet.' |
Generate a single paragraph |
paragraphs($nb = 3, $asText = false) |
array|string |
array($paragraph1, $paragraph2, $paragraph3) |
Generate an array of paragraphs |
text($maxNbChars = 200) |
string |
'Sapiente sunt omnis. Ut pariatur ad autem ducimus et. Voluptas rem voluptas sint modi dolorem amet.' |
Generate a text string. |
Provider/Medical.php
Method | Type | Example | Description |
---|---|---|---|
bloodType(): string |
'AB' |
||
bloodRh(): string |
'+' |
||
bloodGroup(): string |
'AB+' |
Provider/Miscellaneous.php
Method | Type | Example | Description |
---|---|---|---|
boolean($chanceOfGettingTrue = 50) |
bool |
true |
Return a boolean, true or false. |
md5() |
string |
'cfcd208495d565ef66e7dff9f98764da' |
|
sha1() |
string |
'b5d86317c2a144cd04d0d7c03b2b02666fafadf2' |
|
sha256() |
string |
'85086017559ccc40638fcde2fecaf295e0de7ca51b7517b6aebeaaf75b4d4654' |
|
locale() |
string |
'fr_FR' |
|
countryCode() |
string |
'FR' |
|
countryISOAlpha3() |
string |
'FRA' |
|
languageCode() |
string |
'fr' |
|
currencyCode() |
string |
'EUR' |
|
emoji() |
string |
Returns an Emoji (Unicode character between U+1F600 and U+1F637). |
Provider/Payment.php
Method | Type | Example | Description |
---|---|---|---|
creditCardType() |
string Returns a credit card vendor name |
'MasterCard' |
|
creditCardNumber($type = null, $formatted = false, $separator = '-') |
string |
'4485480221084675' |
Returns the String of a credit card number. |
creditCardExpirationDate($valid = true) |
DateTime |
04/13 |
|
creditCardExpirationDateString($valid = true, $expirationDateFormat = null) |
string |
'04/13' |
|
creditCardDetails($valid = true) |
array |
||
iban($countryCode = null, $prefix = '', $length = null) |
string |
||
swiftBicNumber() |
string Swift/Bic number |
'RZTIAT22263' |
Return the String of a SWIFT/BIC number |
Provider/Person.php
Method | Type | Example | Description |
---|---|---|---|
name($gender = null) |
string |
'John Doe' |
|
firstName($gender = null) |
string |
'John' |
|
firstNameMale() |
string |
||
firstNameFemale() |
string |
||
lastName() |
string |
'Doe' |
|
title($gender = null) |
string |
'Mrs.' |
|
titleMale() |
string |
'Mr.' |
|
titleFemale() |
string |
'Mrs.' |
Provider/PhoneNumber.php
Method | Type | Example | Description |
---|---|---|---|
phoneNumber() |
string |
'555-123-546' |
|
e164PhoneNumber() |
string |
+11134567890 |
|
imei() |
int $imei |
'720084494799532' |
Provider/Text.php
Method | Type | Example | Description |
---|---|---|---|
realText($maxNbChars = 200, $indexSize = 2) |
string |
'Alice, swallowing down her flamingo, and began by taking the little golden key' |
Generate a text string by the Markov chain algorithm. |
realTextBetween($minNbChars = 160, $maxNbChars = 200, $indexSize = 2) |
string |
'Alice, swallowing down her flamingo, and began by taking the little golden key' |
Generate a text string by the Markov chain algorithm. |
Provider/UserAgent.php
Method | Type | Example | Description |
---|---|---|---|
macProcessor() |
string |
Generate mac processor | |
linuxProcessor() |
string |
Generate linux processor | |
userAgent() |
string |
'Mozilla/5.0 (Windows CE) AppleWebKit/5350 (KHTML, like Gecko) Chrome/13.0.888.0 Safari/5350' |
Generate a random user agent |
chrome() |
string |
'Mozilla/5.0 (Macintosh; PPC Mac OS X 10_6_5) AppleWebKit/5312 (KHTML, like Gecko) Chrome/14.0.894.0 Safari/5312' |
Generate Chrome user agent |
firefox() |
string |
'Mozilla/5.0 (X11; Linuxi686; rv:7.0) Gecko/20101231 Firefox/3.6' |
Generate Firefox user agent |
safari() |
string |
'Mozilla/5.0 (Macintosh; U; PPC Mac OS X 10_7_1 rv:3.0; en-US) AppleWebKit/534.11.3 (KHTML, like Gecko) Version/4.0 Safari/534.11.3' |
Generate Safari user agent |
opera() |
string |
'Opera/8.25 (Windows NT 5.1; en-US) Presto/2.9.188 Version/10.00' |
Generate Opera user agent |
internetExplorer() |
string |
'Mozilla/5.0 (compatible; MSIE 7.0; Windows 98; Win 9x 4.90; Trident/3.0)' |
Generate Internet Explorer user agent |
windowsPlatformToken() |
string |
||
macPlatformToken() |
string |
||
linuxPlatformToken() |
string |
Provider/Uuid.php
Method | Type | Example | Description |
---|---|---|---|
uuid() |
string |
'7e57d004-2b97-0e7a-b45f-5387367791cd' |
Generate name based md5 UUID (version 3). |
Provider/ja_JP/Address.php
Method | Type | Example | Description |
---|---|---|---|
postcode1() |
111 |
||
postcode2() |
2222 |
||
postcode() |
1112222 |
||
prefecture() |
'東京都' |
||
ward() |
'北区' |
||
areaNumber() |
int |
||
buildingNumber() |
|||
secondaryAddress() |
Provider/ja_JP/Company.php
Method | Type | Example | Description |
---|---|---|---|
companyPrefix() |
Provider/ja_JP/Internet.php
Method | Type | Example | Description |
---|---|---|---|
lastNameAscii() |
|||
firstNameAscii() |
|||
userName() |
'suzuki.taro' |
||
domainName() |
'yamada.jp' |
Provider/ja_JP/Person.php
Method | Type | Example | Description |
---|---|---|---|
kanaName($gender = null) |
string |
'アオタ アキラ' |
|
firstKanaName($gender = null) |
string |
'アキラ' |
|
firstKanaNameMale() |
'アキラ' |
||
firstKanaNameFemale() |
'アケミ' |
||
lastKanaName() |
'アオタ' |