【WordPress入門】アクションフック・フィルターフックを使い倒して WordPressの管理画面をカスタマイズする
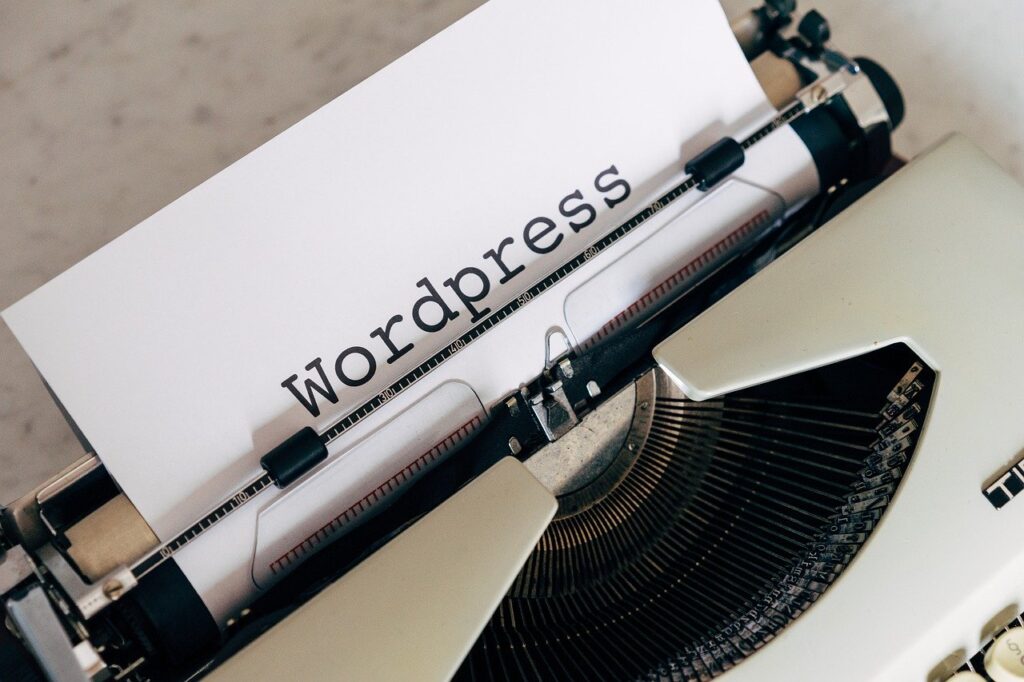
「【WordPress入門】アクションフックとフィルターフックを使いこなそう」で WordPress で主に使用するアクションフックとフィルターフックの一覧を紹介しましたが、今回はそれらのフックを使って WordPress の管理画面をカスタマイズしたいと思います。
目次
- 1 カスタム投稿タイプでブロックエディタを無効化する.
- 2 固定ページの編集画面でビジュアルエディタを禁止する
- 3 管理画面で「投稿」を非表示にする
- 4 管理画面のカスタム投稿タイプの一覧表示でアイキャッチ画像を表示させ、コメント欄を非表示にする
- 5 固定ページの一覧表示でスラッグとページパスを表示する
- 6 管理画面の一覧表示にスタイルを注入する
- 7 新規投稿・投稿編集時に何らかの処理をしたい
- 8 テーマカスタマイザーの設定項目を非表示にする
- 9 投稿内容が半角ひらがなを含む場合にプレビュー画面でハイライト表示する
- 10 投稿のタイトルがセットされていない場合にエラーを表示させる
- 11 メディアの代替テキストがセットされていないときに警告を表示する
- 12 投稿アクションの編集、クイック編集、表示を無効化する.
- 13 クラシックエディタの投稿内容を編集する textarea 要素を変更する
カスタム投稿タイプでブロックエディタを無効化する.
投稿の編集にブロックエディタを利用するかどうかは use_block_editor_for_post
フックを利用します。例えば your_custom_post_type
というカスタム投稿タイプでブロックエディタを無効化する場合は次のようになります。
<?php
add_filter(
'use_block_editor_for_post',
function ( bool $use_block_editor, WP_Post $post ): bool {
return 'your_custom_post_type' === $post->post_type ? false : $use_block_editor;
},
10,
2
);
固定ページの編集画面でビジュアルエディタを禁止する
制作現場では固定ページに関しては HTML を直に書くことがあると思います。このような場合に固定ページの編集画面でビジュアルエディタを無効化するには user_can_richedit
フックを利用します。
<?php
add_filter(
'user_can_richedit',
function( bool $wp_rich_edit ): bool {
return 'page' === get_post_type() ? false : $wp_rich_edit;
}
);
ここでは投稿タイプが page
、つまり固定ページの場合に false
を返してビジュアルエディタを無効化しています。
管理画面で「投稿」を非表示にする
カスタム投稿をメインで利用するようなサイトの場合、デフォルトの「投稿」が不要になることがあります。これを管理画面の左サイドメニューで表示させないようにしたい場合は、admin_menu
フックを利用します。
<?php
add_action(
'admin_menu',
function() {
global $menu;
unset( $menu[5] );
}
);
この方法だとサイドメニューに表示されないだけで、/wp-admin/edit.php
に直接アクセスすると投稿一覧の表示、編集などが可能です。もし編集等もできないようにしたいのであれば、register_post_type_args フックを利用して、register_post_type
に渡す第2引数の public
キーの値を false
にセットしてください。
<?php
add_filter(
'register_post_type_args',
function ( array $args, string $post_type ) {
if ( 'post' === $post_type ) {
$args['public'] = false;
}
return $args;
},
10,
2
);
管理画面のカスタム投稿タイプの一覧表示でアイキャッチ画像を表示させ、コメント欄を非表示にする
管理画面の一覧表示では、デフォルトではタイトル、コメント、日付が表示されます。ここに何か別の項目を追加したい場合は manage_XXXXXX_posts_columns
フックと manage_XXXXXX_posts_custom_column
フックを利用します。ここで XXXXXX
にはカスタム投稿タイプのスラッグ名が入ります。例えばコメント欄を非表示にし、アイキャッチ画像をタイトルの前に表示させる場合は次のようになります。
<?php
add_filter(
'manage_XXXXXX_posts_columns' ,
function( array $columns ): array {
// コメント欄を非表示にする.
unset( $columns['comments'] );
$new_columns = [];
foreach ( $columns as $column_name => $column_display_name ):
if ( $column_name == 'title' ):
$new_columns['thumbnail'] = __('Thumbnail');
endif;
$new_columns[ $column_name ] = $column_display_name;
endforeach;
return $new_columns;
}
);
add_action(
'manage_XXXXXX_posts_custom_column' ,
function( string $column, int $post_id ) {
switch ( $column ) {
case 'thumbnail':
$thumbnail = get_the_post_thumbnail( $post_id, 'medium' );
if ( isset( $thumbnail ) && $thumbnail ):
echo $thumbnail;
else:
echo __('No thumbnail');
endif;
break;
}
},
10,
2
);
add_filter( 'manage_XXXXXX_posts_columns, ...)
の方では一覧のカラムにサムネイル用のカラムを追加しています。そして add_action( 'manage_XXXXXX_posts_custom_column', ...)
の方で、そのカラムに何を echo
させるかを記述しています。ここではアイキャッチ画像を表示するようにしていますが、add_action( 'manage_XXXXXX_posts_custom_column', ...)
で指定する関数の引数には $post_id
が渡ってくるため、カスタムフィールドをはじめとした様々な情報を表示させることができます。Advanced Custom Field などのプラグインを利用してカスタムフィールドを設定している場合は、そのフィールドを表示させるようにしておくと、投稿を編集するユーザーの利便性を上げることができます。
固定ページの一覧表示でスラッグとページパスを表示する
固定ページを作成していると、スラッグやページパスをページ一覧から確認したい場合があります。デフォルトでは「クイック編集」をクリックしないとスラッグを確認できませんが、manage_pages_columns
フックと manage_pages_custom_column
フックに次のような関数を登録することで、一覧に表示させることができます。
<?php
add_filter(
'manage_pages_columns' ,
function( array $columns ): array {
$new_columns = [];
foreach ( $columns as $column_name => $column_display_name ):
$new_columns[ $column_name ] = $column_display_name;
if ( $column_name == 'title' ):
$new_columns['slug'] = 'スラッグ';
$new_columns['path'] = 'ページパス';
endif;
endforeach;
return $new_columns;
}
);
add_action(
'manage_pages_custom_column' ,
function( string $column, int $post_id ) {
switch ( $column ) {
case 'slug':
echo get_post( $post_id )->post_name;
break;
case 'path':
$ancestors = array();
$current_post_id = $post_id;
while ( $current_post_id !== 0 ):
array_unshift( $ancestors, $current_post_id );
$current_post_id = wp_get_post_parent_id( get_post( $current_post_id ) );
endwhile;
$path = array_reduce( $ancestors, function( $carry, $id ) {
return $carry . '/' . get_post( $id )->post_name;
}, '' );
echo '<a href="' . esc_attr( home_url() . $path ) . '" target="_blank">' . esc_attr( $path ) . '</a>';
break;
}
},
10,
2
);
管理画面の一覧表示にスタイルを注入する
manage_XXXXXX_posts_columns
や manage_pages_columns
フックでカラムを追加したときに、その幅を変更したいことがあると思います。こうした場合には、admin_enqueue_scripts
フックでスタイルを注入することができます。例えば上の例のようにアイキャッチ画像を追加した場合には、次のようにするとアイキャッチ画像の幅を 150px にすることができます。
<?php
add_action(
'admin_enqueue_scripts',
function() {
echo '<style>
.column-thumbnail {
width: 150px;
}
.column-thumbnail img {
max-width: 100%;
height: auto;
max-height: 150px;
object-fit: contain;
}
</style>'.PHP_EOL;
}
);
一覧テーブルでは column-[カラム名]
というクラスが各カラムに付与されるため、上記のスラッグやページパスを追加する例の場合には .column-slug
や .column-path
というセレクタに対して CSS を書くことで、カラムのスタイルを上書きすることができます。
新規投稿・投稿編集時に何らかの処理をしたい
新規投稿時、投稿編集時に主役となる関数は wp-includes/post.php
で定義されている wp_insert_post
関数です。ここにはいくつかのアクションフック・フィルターフックが用意されています。これらをコメント文と前後の制御構文とともに抜き出してみます。
<?php
// wp-includes/post.php でのフィルター・アクション
...
/**
* Filters whether the post should be considered "empty".
*
* The post is considered "empty" if both:
* 1. The post type supports the title, editor, and excerpt fields
* 2. The title, editor, and excerpt fields are all empty
*
* Returning a truthy value to the filter will effectively short-circuit
* the new post being inserted, returning 0. If $wp_error is true, a WP_Error
* will be returned instead.
*
* @since 3.3.0
*
* @param bool $maybe_empty Whether the post should be considered "empty".
* @param array $postarr Array of post data.
*/
if ( apply_filters( 'wp_insert_post_empty_content', $maybe_empty, $postarr ) ) {
...
}
...
/**
* Filters the post parent -- used to check for and prevent hierarchy loops.
*
* @since 3.1.0
*
* @param int $post_parent Post parent ID.
* @param int $post_ID Post ID.
* @param array $new_postarr Array of parsed post data.
* @param array $postarr Array of sanitized, but otherwise unmodified post data.
*/
$post_parent = apply_filters( 'wp_insert_post_parent', $post_parent, $post_ID, compact( array_keys( $postarr ) ), $postarr );
...
if ( 'attachment' === $post_type ) {
/**
* Filters attachment post data before it is updated in or added to the database.
*
* @since 3.9.0
*
* @param array $data An array of sanitized attachment post data.
* @param array $postarr An array of unsanitized attachment post data.
*/
$data = apply_filters( 'wp_insert_attachment_data', $data, $postarr );
} else {
/**
* Filters slashed post data just before it is inserted into the database.
*
* @since 2.7.0
*
* @param array $data An array of slashed post data.
* @param array $postarr An array of sanitized, but otherwise unmodified post data.
*/
$data = apply_filters( 'wp_insert_post_data', $data, $postarr );
}
...
if ( $update ) {
/**
* Fires immediately before an existing post is updated in the database.
*
* @since 2.5.0
*
* @param int $post_ID Post ID.
* @param array $data Array of unslashed post data.
*/
do_action( 'pre_post_update', $post_ID, $data );
...
}
...
if ( 'attachment' !== $postarr['post_type'] ) {
wp_transition_post_status( $data['post_status'], $previous_status, $post );
} else {
if ( $update ) {
/**
* Fires once an existing attachment has been updated.
*
* @since 2.0.0
*
* @param int $post_ID Attachment ID.
*/
do_action( 'edit_attachment', $post_ID );
$post_after = get_post( $post_ID );
/**
* Fires once an existing attachment has been updated.
*
* @since 4.4.0
*
* @param int $post_ID Post ID.
* @param WP_Post $post_after Post object following the update.
* @param WP_Post $post_before Post object before the update.
*/
do_action( 'attachment_updated', $post_ID, $post_after, $post_before );
} else {
/**
* Fires once an attachment has been added.
*
* @since 2.0.0
*
* @param int $post_ID Attachment ID.
*/
do_action( 'add_attachment', $post_ID );
}
return $post_ID;
}
if ( $update ) {
/**
* Fires once an existing post has been updated.
*
* @since 1.2.0
*
* @param int $post_ID Post ID.
* @param WP_Post $post Post object.
*/
do_action( 'edit_post', $post_ID, $post );
$post_after = get_post($post_ID);
/**
* Fires once an existing post has been updated.
*
* @since 3.0.0
*
* @param int $post_ID Post ID.
* @param WP_Post $post_after Post object following the update.
* @param WP_Post $post_before Post object before the update.
*/
do_action( 'post_updated', $post_ID, $post_after, $post_before);
}
/**
* Fires once a post has been saved.
*
* The dynamic portion of the hook name, `$post->post_type`, refers to
* the post type slug.
*
* @since 3.7.0
*
* @param int $post_ID Post ID.
* @param WP_Post $post Post object.
* @param bool $update Whether this is an existing post being updated or not.
*/
do_action( "save_post_{$post->post_type}", $post_ID, $post, $update );
/**
* Fires once a post has been saved.
*
* @since 1.5.0
*
* @param int $post_ID Post ID.
* @param WP_Post $post Post object.
* @param bool $update Whether this is an existing post being updated or not.
*/
do_action( 'save_post', $post_ID, $post, $update );
/**
* Fires once a post has been saved.
*
* @since 2.0.0
*
* @param int $post_ID Post ID.
* @param WP_Post $post Post object.
* @param bool $update Whether this is an existing post being updated or not.
*/
do_action( 'wp_insert_post', $post_ID, $post, $update );
さらに wp_transition_post_status
関数では3つのアクションフックが用意されています。
<?php
// wp-includes/post.php で定義されている wp_transition_post_status 関数
/**
* Fires actions related to the transitioning of a post's status.
*
* When a post is saved, the post status is "transitioned" from one status to another,
* though this does not always mean the status has actually changed before and after
* the save. This function fires a number of action hooks related to that transition:
* the generic {@see 'transition_post_status'} action, as well as the dynamic hooks
* {@see '$old_status_to_$new_status'} and {@see '$new_status_$post->post_type'}. Note
* that the function does not transition the post object in the database.
*
* For instance: When publishing a post for the first time, the post status may transition
* from 'draft' – or some other status – to 'publish'. However, if a post is already
* published and is simply being updated, the "old" and "new" statuses may both be 'publish'
* before and after the transition.
*
* @since 2.3.0
*
* @param string $new_status Transition to this post status.
* @param string $old_status Previous post status.
* @param WP_Post $post Post data.
*/
function wp_transition_post_status( $new_status, $old_status, $post ) {
/**
* Fires when a post is transitioned from one status to another.
*
* @since 2.3.0
*
* @param string $new_status New post status.
* @param string $old_status Old post status.
* @param WP_Post $post Post object.
*/
do_action( 'transition_post_status', $new_status, $old_status, $post );
/**
* Fires when a post is transitioned from one status to another.
*
* The dynamic portions of the hook name, `$new_status` and `$old status`,
* refer to the old and new post statuses, respectively.
*
* @since 2.3.0
*
* @param WP_Post $post Post object.
*/
do_action( "{$old_status}_to_{$new_status}", $post );
/**
* Fires when a post is transitioned from one status to another.
*
* The dynamic portions of the hook name, `$new_status` and `$post->post_type`,
* refer to the new post status and post type, respectively.
*
* Please note: When this action is hooked using a particular post status (like
* 'publish', as `publish_{$post->post_type}`), it will fire both when a post is
* first transitioned to that status from something else, as well as upon
* subsequent post updates (old and new status are both the same).
*
* Therefore, if you are looking to only fire a callback when a post is first
* transitioned to a status, use the {@see 'transition_post_status'} hook instead.
*
* @since 2.3.0
*
* @param int $post_id Post ID.
* @param WP_Post $post Post object.
*/
do_action( "{$new_status}_{$post->post_type}", $post->ID, $post );
}
ここまでのフックで、主に使用するのは以下のフックになると思います。
- データベースへの保存前
wp_insert_post_data
– 投稿がデータベースに挿入される前に投稿データをフィルターするフックwp_insert_attachment_data
–wp_insert_post_data
のメディア版pre_post_update
– すでに存在している投稿がデータベースで更新される直前に発火するアクション
- データベースへの保存後
transition_post_status
– 投稿がある状態から別の状態へ遷移したときに発火するアクション{$old_status}_to_{$new_status}
– 投稿がある状態から別の状態へ遷移したときに発火するアクション{$new_status}_{$post->post_type}
– 投稿がある状態から別の状態へ遷移したときに発火するアクションedit_attachment
– 存在しているメディアが更新されたら発火するアクションattachment_updated
– 存在しているメディアが更新されたら発火するアクションadd_attachment
– メディアが新規に追加された直後に発火するアクションedit_post
– すでに存在している投稿(メディアを含む)が更新されたら発火するアクションpost_updated
– すでに存在している投稿(メディアを含む)が更新されたら発火するアクションsave_post_{$post->post_type}
– 投稿(メディアを含む)が保存されたら発火するアクションsave_post
– 投稿(メディアを含む)が保存されたら発火するアクションwp_insert_post
– 投稿(メディアを含む)が保存されたら発火するアクション
save_post_{$post->post_type}
、save_post
、wp_insert_post
はどれも同じ引数を取り順番に呼ばれるため、どれを使用しても同じ結果になります。ただし v3.7.0 から追加された save_post_{$post->post_type}
は投稿タイプで限定されるため、フック関数内で if
などによる制御構文を書かずに済むという利点があります。特に理由がない限りはこのアクションにフックさせるのが無難だと思われます。
例えば book というカスタム投稿タイプの新規投稿・投稿編集時に追加の処理をしたい場合は
<?php
add_action( 'save_post_book', function( $post_ID, $post, $update ) {
// ここで追加の処理を行う
} );
のように関数をフックさせます。
テーマカスタマイザーの設定項目を非表示にする
テーマカスタマイザーで設定項目を加える際に、デフォルトの項目を非表示にしたいことがあります。この場合は customize_register
フックを利用します。
<?php
add_action(
'customize_register',
function( $wp_customize ) {
$wp_customize->remove_section( 'title_tagline' );
$wp_customize->remove_section( 'colors' );
$wp_customize->remove_section( 'header_image' );
$wp_customize->remove_section( 'background_image' );
$wp_customize->remove_section( 'static_front_page');
$wp_customize->remove_section( 'custom_css');
$wp_customize->remove_section( 'add_menu');
remove_action( 'customize_register', array( $wp_customize->nav_menus, 'customize_register' ), 11 );
}
);
投稿内容が半角ひらがなを含む場合にプレビュー画面でハイライト表示する
WordPress ではプレビュー画面かどうかを判定するために is_preview
という関数が用意されています。この関数を the_content
フィルター内で使用することで、投稿本文が半角のひらがなを含む場合にそれをハイライト表示することができます。
add_filter(
'the_content',
function( string $content ): string {
if ( is_preview() ) {
$content = preg_replace( '/([ヲ-゚]+)/u', '<span style="background:yellow">$1</span>', $content );
}
return $content;
}
);
全角英数字を含む場合もハイライト表示させたい場合は、次のようにすることで実現できます。
add_filter(
'the_content',
function( string $content ): string {
if ( is_preview() ) {
$content = preg_replace( '/([ヲ-゚0-9A-Za-z]+)/u', '<span style="background:yellow">$1</span>', $content );
}
return $content;
}
);
さらに機種依存文字についてもハイライト表示させたい場合は次のようになります。
add_filter(
'the_content',
function( string $content ): string {
if ( is_preview() ) {
$content = preg_replace( '/([ヲ-゚0-9A-Za-z①②③④⑤⑥⑦⑧⑨⑩⑪⑫⑬⑭⑯⑰⑱⑲⑳ⅠⅡⅢⅣⅤⅥⅦⅧⅨⅩ㍉㌔㌢㍍㌘㌧㌃㌶㍑㍗㌍㌦㌣㌫㍊㌻㎜㎝㎞㎎㎏㏄㎡㍻〝〟№㏍℡㊤㊥㊦㊧㊨㈱㈲㈹㍾㍽㍼∮∟⊿纊褜鍈銈蓜俉炻昱棈鋹曻彅丨仡仼伀伃伹佖侒侊侚侔俍偀倢俿倞偆偰偂傔僴僘兊兤冝冾凬刕劜劦勀勛匀匇匤卲厓厲叝﨎咜咊咩哿喆坙坥垬埈埇﨏塚增墲夋奓奛奝奣妤妺孖寀甯寘寬尞岦岺峵崧嵓﨑嵂嵭嶸嶹巐弡弴彧德忞恝悅悊惞惕愠惲愑愷愰憘戓抦揵摠撝擎敎昀昕昻昉昮昞昤晥晗晙晴晳暙暠暲暿曺朎朗杦枻桒柀栁桄棏﨓楨﨔榘槢樰橫橆橳橾櫢櫤毖氿汜沆汯泚洄涇浯涖涬淏淸淲淼渹湜渧渼溿澈澵濵瀅瀇瀨炅炫焏焄煜煆煇凞燁燾犱犾猤猪獷玽珉珖珣珒琇珵琦琪琩琮瑢璉璟甁畯皂皜皞皛皦益睆劯砡硎硤礰礼神祥禔福禛竑竧靖竫箞精絈絜綷綠緖繒罇羡羽茁荢荿菇菶葈蒴蕓蕙蕫﨟薰蘒﨡蠇裵訒訷詹誧誾諟諸諶譓譿賰賴贒赶﨣軏﨤逸遧郞都鄕鄧釚釗釞釭釮釤釥鈆鈐鈊鈺鉀鈼鉎鉙鉑鈹鉧銧鉷鉸鋧鋗鋙鋐﨧鋕鋠鋓錥錡鋻﨨錞鋿錝錂鍰鍗鎤鏆鏞鏸鐱鑅鑈閒隆﨩隝隯霳霻靃靍靏靑靕顗顥飼餧館馞驎髙髜魵魲鮏鮱鮻鰀鵰鵫鶴鸙黑ⅰⅱⅲⅳⅴⅵⅶⅷⅸⅹ¬¦'"]+)/u', '<span style="background:yellow">$1</span>', $content );
}
return $content;
}
);
投稿のタイトルがセットされていない場合にエラーを表示させる
投稿のタイトルが空になっていてセットされていない場合に投稿を保存できないようにするには wp_insert_post_empty_content
フィルターを使います。このフィルターは wp-includes/post.php
で定義されている wp_insert_post
関数内で使用されており、投稿の新規作成時や更新時、自動保存時に、投稿のタイトルや内容が空かどうかを確認しています。このフィルター内で投稿内容をチェックし、タイトルが空の場合に wp_die
を呼び出すことで、タイトルがセットされていないときにエラーを表示することができます。
add_filter(
'wp_insert_post_empty_content',
function( bool $maybe_empty, array $postarr ): bool {
if ( '' === $postarr['post_title'] ) {
wp_die( 'タイトルが空になっています。' );
return false;
}
return $maybe_empty;
},
10,
2
);
メディアの代替テキストがセットされていないときに警告を表示する
WordPress のメディアライブラリにアップロードした添付ファイルは、メディアマネージャーで代替テキストやタイトル、キャプションなどを設定することができます。メディアマネージャーは wp-includes/media-template.php
で定義されている wp_print_media_templates
関数で出力されます。この関数の最後に print_media_templates
というアクションフックが用意されているため、メディアマネージャーをカスタマイズしたい場合はこのアクションフックを利用することになります。
ここでは代替テキスト入力用の input 要素に対して placeholder
属性をもたせて、:placeholder-shown
擬似クラスを利用して入力欄が空の場合(つまりプレースホルダーが表示されている場合)に before 擬似要素をもたせることで警告を表示させています。
add_action(
'print_media_templates',
function() {
$html = <<<HTML
<style>span:has(#attachment-details-two-column-alt-text:placeholder-shown):before {
content: "代替テキスト(ALT)が設定されていません。";
display: block;
width: 100%;
color: red;
font-weight: bold;
text-align: right;
}</style>
<script>
function addPlaceholder() {
const input = document.getElementById('attachment-details-two-column-alt-text');
if (input && !input.hasAttribute('placeholder')) input.setAttribute('placeholder', ' ');
setTimeout(addPlaceholder, 1000);
}
window.onload = addPlaceholder;
</script>
HTML;
echo $html;
},
PHP_INT_MAX
);
投稿アクションの編集、クイック編集、表示を無効化する.
WordPress では投稿の一覧画面の各投稿タイトルの下に、クイック編集などのアクションリンクが表示されます。カスタム投稿タイプによっては、これらのアクションリンクを無効化したい場合があると思います。これは post_row_actions
フックを利用することで実現できます。以下の例では「編集」「クイック編集」「表示」を無効化しています。
<?php
add_filter(
'post_row_actions',
function ( array $actions, WP_Post $post ): array {
if ( 'your_custom_post_type' === $post->post_type ) {
unset( $actions['edit'] );
unset( $actions['inline hide-if-no-js'] );
unset( $actions['view'] );
}
return $actions;
},
10,
2
);
クラシックエディタの投稿内容を編集する textarea
要素を変更する
クラシックエディタでは投稿内容を textarea
要素で編集します。これを例えば textarea
要素ではなく input
要素に変更したい場合は、the_editor
フックを利用します。このフックに登録する関数の引数にはエディタを出力するための HTML マークアップが渡されますので、これを input
要素に変更することでテキスト入力欄を変更することができます。以下では 'your_custom_post_type'
というカスタム投稿タイプでエディタを変更しています。
<?php
add_filter(
'the_editor',
function ( string $output ): string {
global $post;
if ( 'your_custom_post_type' === $post->post_type ) {
return '<input id="post-content-input" type="text" readonly value="' . $post->post_content . '" />';
}
return $output;
}
);
これに加えて、wp_editor_settings
フックを使うと、エディタの設定を変更することができます。ここで $settings['editor_css']
に style
要素を渡してやると、上で変更した input
要素にスタイルを当てることができます。
<?php
add_filter(
'wp_editor_settings',
function ( array $settings, string $editor_id ): array {
global $post;
if ( 'your_custom_post_type' === $post->post_type ) {
$settings['_content_editor_dfw'] = false;
$settings['drag_drop_upload'] = false;
$settings['media_buttons'] = false;
$settings['tinymce'] = false;
$settings['quicktags'] = false;
$settings['editor_height'] = 30;
$settings['editor_css'] = '<style>#post-content-input{width:100%;border:1px solid #dcdcde;border-radius:0;margin:40px 0 0;background:#fff;}</style>';
}
return $settings;
},
10,
2
);