【WordPress 入門】 WordPress のテーマ制作で欠かせない関数をまとめてみた
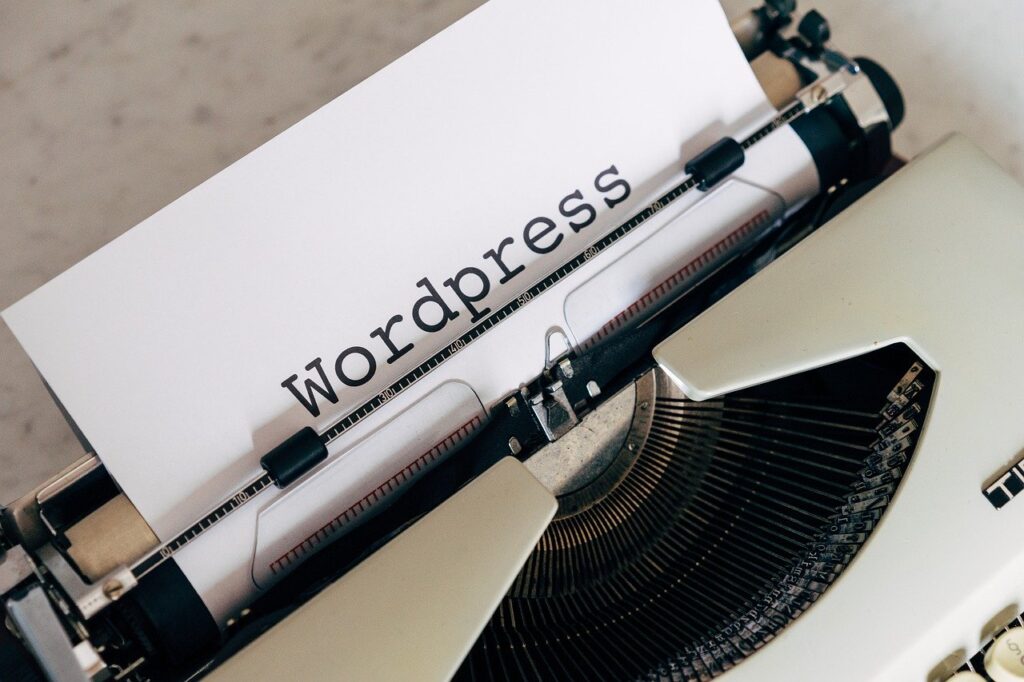
WordPress でテーマ制作する際にいつも調べるのが面倒なので、主に使用する関数をまとめました。
タグ関連
アーカイブページ・投稿ページで投稿につけられたタグ情報を配列として取得します。主にループ内で使用します。
/**
* @param int $id 投稿ID
*/
(array|false|WP_Error) get_the_tags( int $id = 0 )
https://developer.wordpress.org/reference/functions/get_the_tags/
使用例
<?php if ( get_the_tags() ) { ?>
<div class="tags">
<i class="fas fa-tag"></i>
<?php the_tags( '', ',', '' ); ?>
</div>
<?php
}
アーカイブページ・投稿ページで投稿につけられたタグが出力されます。主にループ内で使用します。
/**
* @param string $before リストの最初に入れるHTML/文字
* @param string $sep 各タグの間に入れるHTML/文字
* @param string $after リストの最後に入れるHTML/文字
*/
void the_tags( string $before = null, string $sep = ', ', string $after = '' )
https://developer.wordpress.org/reference/functions/the_tags/
使用例
<?php if ( get_the_tags() ) { ?>
<div class="tags">
<i class="fas fa-tag"></i>
<?php the_tags( '', ',', '' ); ?>
</div>
<?php
}
出力例
上記の使用例の場合には以下のようなHTMLが出力されます。
<div class="tags">
<i class="fas fa-tag"></i>
<a href="..." rel="tag">タグ名1</a>,<a href="..." rel="tag">タグ名2</a>
</div>
the_tags( '<ul><li>', '</li><li>', '</li></ul>' );
のようにするとリスト要素で囲むことができます:
<ul>
<li><a href="..." rel="tag">タグ名1</a></li>
<li><a href="..." rel="tag">タグ名2</a></li>
</ul>
カテゴリー関連
get_the_category
アーカイブページ・投稿ページで投稿につけられたカテゴリー情報を配列として取得します。主にループ内で使用します。関数内では get_the_terms( $id, 'category' )
が実行されているため、この関数で取得できるのはデフォルトのカテゴリーのみです。カスタムタクソノミーについては get_the_terms
関数を使用します。
/**
* @param int $id 投稿ID
*/
(array|false|WP_Error) get_the_category( int $id = 0 )
https://developer.wordpress.org/reference/functions/get_the_category/
使用例
<?php if (get_the_category()) { ?>
<div class="tags">
<i class="fas fa-tag"></i>
<?php the_tags('', ',', ''); ?>
</div>
<?php
}
wp_list_categories
登録されているカテゴリーのリストを取得します。アーカイブページ・投稿ページで使用することで、カテゴリーページへのリンクを表示することができます。
/**
* @param string|array $args オプション引数
*/
(false|string) wp_list_categories( string|array $args = '' )
https://developer.wordpress.org/reference/functions/wp_list_categories/
使用例
wp_list_categories( array(
'title_li' => '',
'hide_empty' => false
) );
日付関連
日付関連の関数については WordPress 5.3 で大きく改善されました。WordPress では長らく WordPress タイムスタンプ(UNIX タイムスタンプ+タイムゾーンオフセット)が使用されてきましたが、バグの温床になっていました。5.3 以降は基本的には UNIX タイムスタンプを利用するような関数だけを利用するように推奨されています。それまで使われてきた get_the_time
や current_time
、date_i18n
は基本的には使用しないようにしてください。
get_post_datetime
投稿が作成、または修正された日時を DateTimeImmutable として取得します。関数の呼び出しに続けて DateTimeImmutable
クラスの format
メソッドを呼び出すことで日付を好きなフォーマットで取得することができます。投稿の日付に関してはこの関数だけで十分です。
/**
* @param int|WP_Post $post 投稿ID、または投稿オブジェクト.
* デフォルト値は `null` ですが、`null` が渡されるとグローバル変数の `$post` に入っている投稿がセットされます.
* @param string $field 作成日/更新日のどちらを返すかを 'date' / 'modified' で指定します.
* デフォルト値は 'date'.
* @param string $source ローカル/UTC 時刻のどちらを返すかを 'local' / 'gmt' で指定します.
* デフォルト値は 'local'.
*/
(DateTimeImmutable|false) get_post_datetime( int|WP_Post $post = null, string $field = 'date', string $source = 'local' )
https://developer.wordpress.org/reference/functions/get_post_datetime/
使用例
ループ内で使う場合は第一引数に null
を渡しておけば大丈夫です。
<time class="time" datetime="<?php echo esc_attr( get_post_datetime( null, 'modified' )->format( 'c' ) ); ?>"><?php echo esc_html( get_post_datetime( null, 'modified' )->format( 'Y年m月d日H:i更新' ) ); ?></time>
<time class="time" datetime="<?php echo esc_attr( get_post_datetime()->format( 'c' ) ); ?>"><?php echo esc_html( get_post_datetime()->format( 'Y年m月d日H:i公開' ) ); ?></time>
wp_get_archives
アーカイブへのリンクを出力します。
(string|void) wp_get_archives( string|array $args = '' )
https://developer.wordpress.org/reference/functions/wp_get_archives/
パラメーター
$args
の一覧は Codex を参照してください。主に使用するのは以下のキーです。
パラメータ | 型 | デフォルト | 説明 |
---|---|---|---|
show_post_count |
bool | false |
投稿数を表示するかどうか |
before |
(string) | アーカイブページへのリンクの前に挿入するHTML | |
after |
(string) | アーカイブページへのリンクの後ろに挿入するHTML | |
post_type |
(string) | post |
投稿タイプ |
使用例
wp_list_categories( array(
'title_li' => '',
'hide_empty' => false
) );
アイキャッチ画像(サムネイル画像)関連
get_post_thumbnail_id
アーカイブページ・投稿ページで投稿のアイキャッチ画像の ID を取得します。主にループ内で使用します。取得した ID を wp_get_attachment_image_src
関数に渡すことでアイキャッチ画像の情報を取得することができます。
/**
* @param int|WP_Post $post 投稿ID、もしくは WP_Post オブジェクト.
* デフォルト値は null. null の場合にはグローバル変数の `$post` が指す投稿になります.
*/
(string|int) get_post_thumbnail_id( int|WP_Post $post = null )
https://developer.wordpress.org/reference/functions/get_post_thumbnail_id/
wp_get_attachment_image_src
添付画像のURL、幅、高さ、リサイズされた画像かどうかの情報を取得します。
/**
* @param int $attachment_id 添付画像の ID.
* @param string|array $size 画像のサイズ. 'thumbnail'、'post-thumbnail'、'small'、'medium'、'large'などの文字列や、幅と高さのピクセル値を要素とする配列です.
* @param bool $icon 画像をアイコンとして扱うかどうか.
*/
(false|array) wp_get_attachment_image_src( int $attachment_id, string|array $size = 'thumbnail', bool $icon = false )
https://developer.wordpress.org/reference/functions/wp_get_attachment_image_src/
戻り値
画像が見つかれば、URL、幅、高さ、リサイズされているかどうかの真偽値を要素とする配列が返ります。画像が見つからなければ false
が返ってきます。
使用例
<?php $thumbnail = wp_get_attachment_image_src( get_post_thumbnail_id(), 'full' ); ?>
<amp-img src="<?php echo esc_url( $thumbnail[0] ); ?>" width="<?php echo esc_attr( $thumbnail[1] ); ?>" height="<?php echo esc_attr( $thumbnail[2] ); ?>" layout="responsive"></amp-img>
ナビゲーション関連
アーカイブページで「前の投稿」「次の投稿」のようなナビゲーションが出力されます。4.1.0 から導入されました。ページネーションが必要な場合は the_posts_pagination を利用してください。
/**
* @param array $args {
* @type string $prev_text 前のページへのリンクテキスト.
* デフォルトは 'Older posts'.
* @type string $next_text 次のページへのリンクテキスト.
* デフォルトは 'Newer posts'.
* @type string $screen_reader_text nav要素のためのスクリーンリーダテキスト.
* デフォルトは 'Posts navigation'.
* @type string $aria_label nav要素のためのARIA ラベルテキスト.* デフォルトは 'Posts'.
* @type string $class nav要素に付与するクラス.
* デフォルトは 'posts-navigation'.
* }
*/
void the_posts_navigation( array $args = array() )
https://developer.wordpress.org/reference/functions/the_posts_navigation/
使用例
<?php
the_posts_navigation( array(
'prev_text' => '<',
'next_text' => '>'
) );
出力例
<nav class="navigation posts-navigation" role="navigation">
<h2 class="screen-reader-text">投稿ナビゲーション</h2>
<div class="nav-links">
<div class="nav-previous">
<a href="...">過去の投稿</a>
</div>
<div class="nav-next">
<a href="...">新しい投稿</a>
</div>
</div>
</nav>
the_posts_pagination
アーカイブページで「1」「2」「3」のようなページネーションが出力されます。4.1.0 から導入されました。
void the_posts_pagination( array $args = array() )
https://developer.wordpress.org/reference/functions/the_posts_pagination/
パラメーター
$args
は paginate_links
の引数と同じ連想配列です。主に使用するものは以下です。
パラメータ | 型 | デフォルト | 説明 |
---|---|---|---|
show_all | bool | false | すべてのページを表示するかどうか |
prev_next | bool | true | 前のページ・次のページへのリンクも表示するかどうか |
prev_text | string | __( '« Previous' ) |
前のページへのリンクテキスト |
next_text | string | __( 'Next »' ) |
次のページへのリンクテキスト |
screen_reader_text | string | __( 'Posts navigation' ) |
nav要素のためのスクリーンリーダテキスト |
使用例
<?php
the_posts_pagination( array(
'prev_text' => '<',
'next_text' => '>'
) );
出力例
<nav class="navigation pagination" role="navigation">
<h2 class="screen-reader-text">投稿ナビゲーション</h2>
<div class="nav-links">
<a class="prev page-numbers" href="...">前へ</a>
<a class="page-numbers" href="...">1</a>
<span aria-current="page" class="page-numbers current">2</span>
<a class="page-numbers" href="...">3</a>
<a class="next page-numbers" href="...">次へ</a>
</div>
</nav>
投稿ページで「前の投稿」「次の投稿」のようなナビゲーションが出力されます。4.1.0 から導入されました。
void the_post_navigation( array $args = array() )
https://developer.wordpress.org/reference/functions/the_post_navigation/
パラメーター
$args
は以下のようなキーを含む連想配列になります。
パラメータ | 型 | デフォルト | 説明 |
---|---|---|---|
prev_text | string | '%title' |
前のページへのリンクテキスト |
next_text | string | '%title' |
次のページへのリンクテキスト |
screen_reader_text | string | __( 'Post navigation' ) |
nav要素のためのスクリーンリーダテキスト |
使用例
<?php
the_post_navigation( array(
'prev_text' => '<',
'next_text' => '>'
) );
出力例
<nav class="navigation post-navigation" role="navigation">
<h2 class="screen-reader-text">投稿ナビゲーション</h2>
<div class="nav-links">
<div class="nav-previous">
<a href="..." rel="prev">[前の投稿のタイトル]</a>
</div>
<div class="nav-next">
<a href="..." rel="next">[次の投稿のタイトル]</a>
</div>
</div>
</nav>
ファイル関連
get_theme_file_uri
テーマ内にあるファイルのURIを取得します。4.7.0から導入されました。以前は get_stylesheet_directory_uri
関数でテーマディレクトリへの URI を取得し、相対ファイルパスを文字列として連結することでファイルへの URI を取得していましたが、この関数にテーマディレクトリからの相対ファイルパスを与えることでファイルのURIが取得できるようになりました。
/**
* @param string $file スタイルシートが置かれているディレクトリ内で探すファイル名.
*/
(string) get_theme_file_uri( string $file = '' )
https://developer.wordpress.org/reference/functions/get_theme_file_uri/
get_theme_file_path
テーマ内にあるファイルのパス名を取得します。4.7.0から導入されました。以前は get_stylesheet_directory
関数でテーマディレクトリへのパス名を取得し、相対ファイルパスを文字列として連結することでファイルへのパス名を取得していましたが、この関数にテーマディレクトリからの相対ファイルパスを与えることでファイルのパス名が取得できるようになりました。
/**
* @param string $file スタイルシートが置かれているディレクトリ内で探すファイル名.
*/
(string) get_theme_file_path( string $file = '' )
https://developer.wordpress.org/reference/functions/get_theme_file_path/
JavaScript 関連
wp_enqueue_script
テーマ制作をする際についつい header.php
や footer.php
で JavaScript を直接読み込みたくなりますが、functions.php から wp_enqueue_script
を呼び出してキューに入れるのが WordPress らしい JavaScript の読み込みです。wp_enqueue_scripts
アクションにフックさせて呼び出します。後述する wp_add_inline_script
と合わせると、jQuery のプラグインや Object-fit-images のようなポリフィルの読み込み + 起動をまとめて行うことができます。
/**
* @param string $handle スクリプトの名前. 固有のもの(同じ名前のものが他にない)でなければなりません.
* @param string $src スクリプトの完全な URL、もしくは WordPress のルートディレクトリからの相対パス.
* @param array $deps 依存関係にあるスクリプトの名前.
* @param string|bool|null $ver スクリプトのバージョン.
* @param bool $in_footer スクリプトの読み込み位置. true なら body要素の閉じタグの直前、false なら head 要素内.
*/
void wp_enqueue_script( string $handle, string $src = '', array $deps = array(), string|bool|null $ver = false, bool $in_footer = false )
https://developer.wordpress.org/reference/functions/wp_enqueue_script/
使用例
<?php
add_action( 'wp_enqueue_scripts', function() {
wp_enqueue_script( 'vuejs', 'https://cdn.jsdelivr.net/npm/vue@2.6.10/dist/vue.min.js', array(), '2.6.10', false );
} );
wp_add_inline_script
wp_enqueue_script でエンキューされているスクリプトの名前を指定することで、インラインの JavaScript を埋め込むことができます。wp_enqueue_scripts
アクションにフックさせて呼び出します。よくある使用例としては、jQuery のプラグインを wp_enqueue_script で読み込ませ、プラグインの起動コードをインラインで埋め込むような場合です。
/**
* @param string $handle インラインスクリプトを追加するスクリプトの名前. エンキューされているスクリプト名でなければならない.
* @param string $data インラインで追加するスクリプト.
* @param string $position スクリプトの後に追加するか前に追加するか.
*/
(bool) wp_add_inline_script( string $handle, string $data, string $position = 'after' )
https://developer.wordpress.org/reference/functions/wp_add_inline_script/
使用例
<?php
add_action( 'wp_enqueue_scripts', function() {
wp_enqueue_script( 'object-fit-images', 'https://cdnjs.cloudflare.com/ajax/libs/object-fit-images/3.2.4/ofi.min.js', array(), '3.2.4', false );
wp_add_inline_script( 'object-fit-images', 'jQuery(function () { objectFitImages() });' );
} );