【WordPress 入門】WordPress のプラグイン開発に有用な関数をまとめてみた
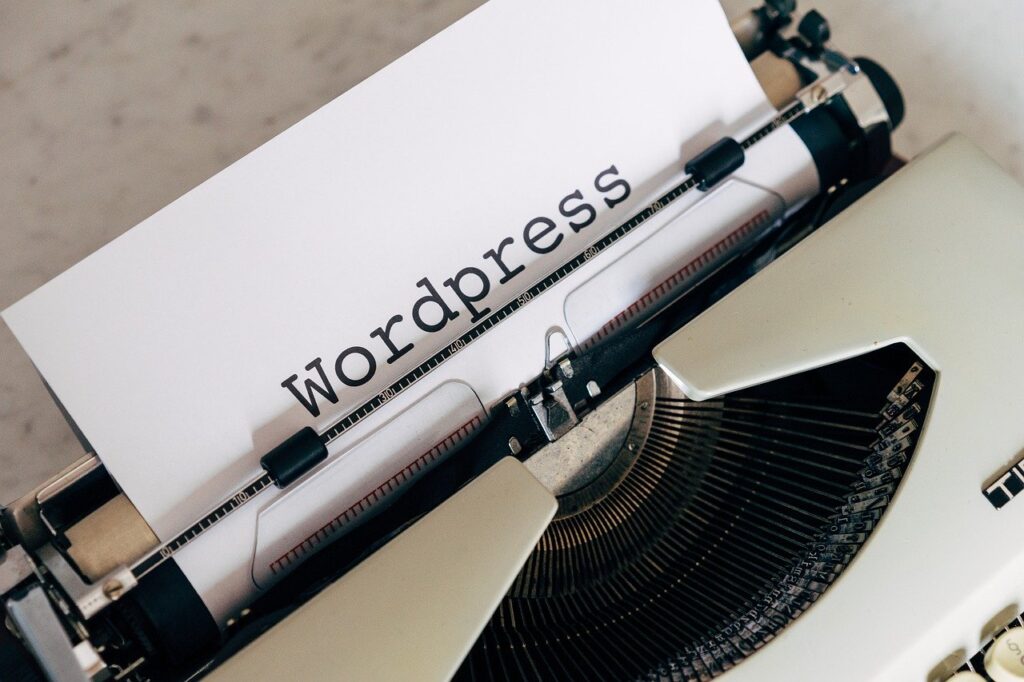
WordPress にはコアで使用されている関数がたくさんあります。これらはプラグインやテーマからも利用することができます。ここではコアで定義されている関数をいくつかピックアップしました。
目次
- 1 配列操作
- 2 クエリ関連
- 3 日付関連
- 4 ユーティリティ関数
- 4.1 __return_true
- 4.2 __return_false
- 4.3 __return_zero
- 4.4 wp_validate_boolean
- 4.5 is_wp_version_compatible
- 4.6 is_php_version_compatible
- 4.7 wp_unique_id
- 4.8 wp_generate_uuid4
- 4.9 wp_is_uuid
- 4.10 is_wp_error
- 4.11 wp_die
- 4.12 maybe_serialize
- 4.13 maybe_unserialize
- 4.14 is_serialized
- 4.15 absint
- 4.16 number_format_i18n
- 4.17 wp_get_referer
- 4.18 get_allowed_mime_types
- 4.19 get_num_queries
- 5 HTTP リクエスト関連
- 6 ファイル・ディレクトリ関連
配列操作
wp_parse_args
第1引数で与えられた配列を第2引数で与えられたデフォルトの配列にマージします。プラグインが用意するデフォルトの値をユーザーが設定した値で上書きするような場合に使います。
/**
* Merge user defined arguments into defaults array.
*
* This function is used throughout WordPress to allow for both string or array
* to be merged into another array.
*
* @since 2.2.0
* @since 2.3.0 `$args` can now also be an object.
*
* @param string|array|object $args Value to merge with $defaults.
* @param array $defaults Optional. Array that serves as the defaults. Default empty.
* @return array Merged user defined values with defaults.
*/
(array) function wp_parse_args( $args, $defaults = '' )
wp_is_numeric_array
数値でインデックスされている配列かどうかを調べます。コアではあまり使われていません。
/**
* Determines if the variable is a numeric-indexed array.
*
* @since 4.4.0
*
* @param mixed $data Variable to check.
* @return bool Whether the variable is a list.
*/
(bool) function wp_is_numeric_array( $data )
wp_array_slice_assoc
連想配列から必要な key-value ペアだけを切り取って返してくれます。
/**
* Extract a slice of an array, given a list of keys.
*
* @since 3.1.0
*
* @param array $array The original array.
* @param array $keys The list of keys.
* @return array The array slice.
*/
(array) function wp_array_slice_assoc( $array, $keys )
wp_filter_object_list
WordPress のコアが用意している便利な関数ですが、あまり知られていないように思います。これはオブジェクト配列から必要なものだけをフィルターすることができます。例えば
wp_filter_object_list(get_posts(), array('ID' => 1))
とすると、get_posts()
で得られる Post オブジェクトの配列から ID が 1 の投稿だけを取得することができます。$operator
では and
, or
, not
が指定できるため、複数の条件すべてにマッチするもの、複数の条件のどれかにマッチするもの、与えた条件にマッチしないものといったフィルタリングが可能です。
また、$field
を指定することで、フィルターするオブジェクトの一部だけを返すことが可能になります。例えば
wp_filter_object_list( get_users(), array( 'roles' => array( 'administrator' ) ), 'and', 'ID' ) );
とすると、管理者権限のユーザーの ID を要素とする配列を取得することができます。
/**
* Filters a list of objects, based on a set of key => value arguments.
*
* @since 3.0.0
* @since 4.7.0 Uses `WP_List_Util` class.
*
* @param array $list An array of objects to filter
* @param array $args Optional. An array of key => value arguments to match
* against each object. Default empty array.
* @param string $operator Optional. The logical operation to perform. 'or' means
* only one element from the array needs to match; 'and'
* means all elements must match; 'not' means no elements may
* match. Default 'and'.
* @param bool|string $field A field from the object to place instead of the entire object.
* Default false.
* @return array A list of objects or object fields.
*/
(array) function wp_filter_object_list( $list, $args = array(), $operator = 'and', $field = false )
wp_list_filter
$field
が指定できない点を除いて wp_filter_object_list
と(実装面でも)同じです。オブジェクトをそのまま欲しい場合はこちらを使えばいいと思います。場面によっては array_filter
で書くよりも短く書くことができるため、覚えておくと便利な関数です。
/**
* Filters a list of objects, based on a set of key => value arguments.
*
* @since 3.1.0
* @since 4.7.0 Uses `WP_List_Util` class.
*
* @param array $list An array of objects to filter.
* @param array $args Optional. An array of key => value arguments to match
* against each object. Default empty array.
* @param string $operator Optional. The logical operation to perform. 'AND' means
* all elements from the array must match. 'OR' means only
* one element needs to match. 'NOT' means no elements may
* match. Default 'AND'.
* @return array Array of found values.
*/
(array) function wp_list_filter( $list, $args = array(), $operator = 'AND' )
wp_list_pluck
オブジェクトの配列から、オブジェクトの一部のプロパティだけを抜き出した配列を生成します。$index_key
を指定すると(指定できるのはオブジェクトがもつプロパティ名です)、$index_key
プロパティの値 => $field
プロパティの値という連想配列が返ってきます。例えば
$filtered = wp_list_pluck( get_posts(), 'post_title', 'ID' );
var_dump( $filtered );
とすると、
array(4) {
[6]=>
string(28) "投稿ID = 6 のタイトル"
[5]=>
string(28) "投稿ID = 5 のタイトル"
[4]=>
string(28) "投稿ID = 4 のタイトル"
[1]=>
string(28) "投稿ID = 1 のタイトル"
}
という連想配列を取得することができます。$index_key
を渡さない場合は、オブジェクト配列を配列に変換してくれるため、使いどころの多い関数だと思います。コアでもあちこちで使われています。
/**
* Pluck a certain field out of each object in a list.
*
* This has the same functionality and prototype of
* array_column() (PHP 5.5) but also supports objects.
*
* @since 3.1.0
* @since 4.0.0 $index_key parameter added.
* @since 4.7.0 Uses `WP_List_Util` class.
*
* @param array $list List of objects or arrays
* @param int|string $field Field from the object to place instead of the entire object
* @param int|string $index_key Optional. Field from the object to use as keys for the new array.
* Default null.
* @return array Array of found values. If `$index_key` is set, an array of found values with keys
* corresponding to `$index_key`. If `$index_key` is null, array keys from the original
* `$list` will be preserved in the results.
*/
(array) function wp_list_pluck( $list, $field, $index_key = null )
wp_parse_list
","、スペースで区切られた文字列を配列に変換します。配列が与えられた場合はそのまま返します。
/**
* Cleans up an array, comma- or space-separated list of scalar values.
*
* @since 5.1.0
*
* @param array|string $list List of values.
* @return array Sanitized array of values.
*/
(array) function wp_parse_list( $list )
wp_parse_id_list
","、スペースで区切られた ID の文字列、もしくは ID の配列から重複したもの、負の値をとるものを取り除いて新しい配列を返します。
/**
* Clean up an array, comma- or space-separated list of IDs.
*
* @since 3.0.0
*
* @param array|string $list List of ids.
* @return array Sanitized array of IDs.
*/
(array) function wp_parse_id_list( $list )
クエリ関連
build_query
(連想)配列から URL クエリを生成します。
/**
* Build URL query based on an associative and, or indexed array.
*
* This is a convenient function for easily building url queries. It sets the
* separator to '&' and uses _http_build_query() function.
*
* @since 2.3.0
*
* @see _http_build_query() Used to build the query
* @link https://secure.php.net/manual/en/function.http-build-query.php for more on what
* http_build_query() does.
*
* @param array $data URL-encode key/value pairs.
* @return string URL-encoded string.
*/
(string) function build_query( $data )
add_query_arg
( $key, $value, $url )
でクエリを追加するか、( arrray, $url )
で連想配列で渡されたクエリを追加します。
/**
* Retrieves a modified URL query string.
*
* You can rebuild the URL and append query variables to the URL query by using this function.
* There are two ways to use this function; either a single key and value, or an associative array.
*
* Using a single key and value:
*
* add_query_arg( 'key', 'value', 'http://example.com' );
*
* Using an associative array:
*
* add_query_arg( array(
* 'key1' => 'value1',
* 'key2' => 'value2',
* ), 'http://example.com' );
*
* Omitting the URL from either use results in the current URL being used
* (the value of `$_SERVER['REQUEST_URI']`).
*
* Values are expected to be encoded appropriately with urlencode() or rawurlencode().
*
* Setting any query variable's value to boolean false removes the key (see remove_query_arg()).
*
* Important: The return value of add_query_arg() is not escaped by default. Output should be
* late-escaped with esc_url() or similar to help prevent vulnerability to cross-site scripting
* (XSS) attacks.
*
* @since 1.5.0
*
* @param string|array $key Either a query variable key, or an associative array of query variables.
* @param string $value Optional. Either a query variable value, or a URL to act upon.
* @param string $url Optional. A URL to act upon.
* @return string New URL query string (unescaped).
*/
(string) function add_query_arg()
remove_query_arg
クエリを削除します。
/**
* Removes an item or items from a query string.
*
* @since 1.5.0
*
* @param string|array $key Query key or keys to remove.
* @param bool|string $query Optional. When false uses the current URL. Default false.
* @return string New URL query string.
*/
(string) function remove_query_arg( $key, $query = false )
日付関連
current_time
現在時刻を取得します。
/**
* Retrieve the current time based on specified type.
*
* The 'mysql' type will return the time in the format for MySQL DATETIME field.
* The 'timestamp' type will return the current timestamp.
* Other strings will be interpreted as PHP date formats (e.g. 'Y-m-d').
*
* If $gmt is set to either '1' or 'true', then both types will use GMT time.
* if $gmt is false, the output is adjusted with the GMT offset in the WordPress option.
*
* @since 1.0.0
*
* @param string $type Type of time to retrieve. Accepts 'mysql', 'timestamp', or PHP date
* format string (e.g. 'Y-m-d').
* @param int|bool $gmt Optional. Whether to use GMT timezone. Default false.
* @return int|string Integer if $type is 'timestamp', string otherwise.
*/
(int|string) function current_time( $type, $gmt = 0 )
date_i18n
ローカル日時を取得することができます。
$date = date_i18n( 'Y年m月d日 H:i:s' );
/**
* Retrieve the date in localized format, based on a sum of Unix timestamp and
* timezone offset in seconds.
*
* If the locale specifies the locale month and weekday, then the locale will
* take over the format for the date. If it isn't, then the date format string
* will be used instead.
*
* @since 0.71
*
* @global WP_Locale $wp_locale
*
* @param string $dateformatstring Format to display the date.
* @param int|bool $timestamp_with_offset Optional. A sum of Unix timestamp and timezone offset in seconds.
* Default false.
* @param bool $gmt Optional. Whether to use GMT timezone. Only applies if timestamp is
* not provided. Default false.
*
* @return string The date, translated if locale specifies it.
*/
(string) function date_i18n( $dateformatstring, $timestamp_with_offset = false, $gmt = false )
human_readable_duration
経過時間をヒューマンリーダブルな形式に変換します。
/**
* Convert a duration to human readable format.
*
* @since 5.1.0
*
* @param string $duration Duration will be in string format (HH:ii:ss) OR (ii:ss),
* with a possible prepended negative sign (-).
* @return string|false A human readable duration string, false on failure.
*/
(string|false) function human_readable_duration( $duration = '' )
ユーティリティ関数
__return_true
/**
* Returns true.
*
* Useful for returning true to filters easily.
*
* @since 3.0.0
*
* @see __return_false()
*
* @return true True.
*/
(bool) function __return_true()
__return_false
当然 false
を返す関数もあります。
/**
* Returns false.
*
* Useful for returning false to filters easily.
*
* @since 3.0.0
*
* @see __return_true()
*
* @return false False.
*/
(bool) function __return_false()
__return_zero
0 を返す関数というのもあります。
/**
* Returns 0.
*
* Useful for returning 0 to filters easily.
*
* @since 3.0.0
*
* @return int 0.
*/
(int) function __return_zero()
wp_validate_boolean
引数を boolean としてフィルター/検証します。やっているのは filter_var( $var, FILTER_VALIDATE_BOOLEAN )
と同じことです。
/**
* Filter/validate a variable as a boolean.
*
* Alternative to `filter_var( $var, FILTER_VALIDATE_BOOLEAN )`.
*
* @since 4.0.0
*
* @param mixed $var Boolean value to validate.
* @return bool Whether the value is validated.
*/
(bool) function wp_validate_boolean( $var )
is_wp_version_compatible
引数で与えられた WP バージョン以上のものがインストールされているかどうかを調べます。プラグインで新しい機能等を使用している場合は、register_activation_hook
内でこの関数を使ってチェックする必要があります。
/**
* Checks compatibility with the current WordPress version.
*
* @since 5.2.0
*
* @param string $required Minimum required WordPress version.
* @return bool True if required version is compatible or empty, false if not.
*/
(bool) function is_wp_version_compatible( $required )
is_php_version_compatible
サーバーで動いている PHP のバージョンが引数で与えられたバージョン以上かどうかをチェックします。これも register_activation_hook
にフックさせる関数内で使用するのがメインだと思います。
/**
* Checks compatibility with the current PHP version.
*
* @since 5.2.0
*
* @param string $required Minimum required PHP version.
* @return bool True if required version is compatible or empty, false if not.
*/
(bool) function is_php_version_compatible( $required )
wp_unique_id
PHP のプロセス内でユニークな ID を発行することができます。ver5.0.3 で追加されましたが、コア内ではまだ使われていません。
/**
* Get unique ID.
*
* This is a PHP implementation of Underscore's uniqueId method. A static variable
* contains an integer that is incremented with each call. This number is returned
* with the optional prefix. As such the returned value is not universally unique,
* but it is unique across the life of the PHP process.
*
* @since 5.0.3
*
* @staticvar int $id_counter
*
* @param string $prefix Prefix for the returned ID.
* @return string Unique ID.
*/
(string) function wp_unique_id( $prefix = '' )
wp_generate_uuid4
ランダムな UUID (version 4) を生成します。
/**
* Generate a random UUID (version 4).
*
* @since 4.7.0
*
* @return string UUID.
*/
(string) wp_generate_uuid4()
wp_is_uuid
有効な UUID かどうかを判別します。
/**
* Validates that a UUID is valid.
*
* @since 4.9.0
*
* @param mixed $uuid UUID to check.
* @param int $version Specify which version of UUID to check against. Default is none,
* to accept any UUID version. Otherwise, only version allowed is `4`.
* @return bool The string is a valid UUID or false on failure.
*/
(bool) function wp_is_uuid( mixed $uuid, int $version = null )
is_wp_error
引数が WP_Error
のインスタンス化どうかを判定します。
/**
* Check whether variable is a WordPress Error.
*
* Returns true if $thing is an object of the WP_Error class.
*
* @since 2.1.0
*
* @param mixed $thing Check if unknown variable is a WP_Error object.
* @return bool True, if WP_Error. False, if not WP_Error.
*/
(bool) function is_wp_error( $thing )
wp_die
WordPress の実行を停止してエラーメッセージを HTML に表示します。プラグインで必要な定数が wp-config.php で定義されていないような場合に使うことがあります。
/**
* Kills WordPress execution and displays HTML page with an error message.
*
* This function complements the `die()` PHP function. The difference is that
* HTML will be displayed to the user. It is recommended to use this function
* only when the execution should not continue any further. It is not recommended
* to call this function very often, and try to handle as many errors as possible
* silently or more gracefully.
*
* As a shorthand, the desired HTTP response code may be passed as an integer to
* the `$title` parameter (the default title would apply) or the `$args` parameter.
*
* @since 2.0.4
* @since 4.1.0 The `$title` and `$args` parameters were changed to optionally accept
* an integer to be used as the response code.
* @since 5.1.0 The `$link_url`, `$link_text`, and `$exit` arguments were added.
*
* @global WP_Query $wp_query Global WP_Query instance.
*
* @param string|WP_Error $message Optional. Error message. If this is a WP_Error object,
* and not an Ajax or XML-RPC request, the error's messages are used.
* Default empty.
* @param string|int $title Optional. Error title. If `$message` is a `WP_Error` object,
* error data with the key 'title' may be used to specify the title.
* If `$title` is an integer, then it is treated as the response
* code. Default empty.
* @param string|array|int $args {
* Optional. Arguments to control behavior. If `$args` is an integer, then it is treated
* as the response code. Default empty array.
*
* @type int $response The HTTP response code. Default 200 for Ajax requests, 500 otherwise.
* @type string $link_url A URL to include a link to. Only works in combination with $link_text.
* Default empty string.
* @type string $link_text A label for the link to include. Only works in combination with $link_url.
* Default empty string.
* @type bool $back_link Whether to include a link to go back. Default false.
* @type string $text_direction The text direction. This is only useful internally, when WordPress
* is still loading and the site's locale is not set up yet. Accepts 'rtl'.
* Default is the value of is_rtl().
* @type string $code Error code to use. Default is 'wp_die', or the main error code if $message
* is a WP_Error.
* @type bool $exit Whether to exit the process after completion. Default true.
* }
*/
(bool) function wp_die( $message = '', $title = '', $args = array() )
maybe_serialize
引数で与えた文字列/配列/オブジェクトをシリアライズします。
/**
* Serialize data, if needed.
*
* @since 2.0.5
*
* @param string|array|object $data Data that might be serialized.
* @return mixed A scalar data
*/
(mixed) function maybe_serialize( $data )
maybe_unserialize
アンシリアライズします。
/**
* Unserialize value only if it was serialized.
*
* @since 2.0.0
*
* @param string $original Maybe unserialized original, if is needed.
* @return mixed Unserialized data can be any type.
*/
(mixed) function maybe_unserialize( $original )
is_serialized
シリアライズされているかどうかをチェックします。
/**
* Check value to find if it was serialized.
*
* If $data is not an string, then returned value will always be false.
* Serialized data is always a string.
*
* @since 2.0.5
*
* @param string $data Value to check to see if was serialized.
* @param bool $strict Optional. Whether to be strict about the end of the string. Default true.
* @return bool False if not serialized and true if it was.
*/
(bool) function is_serialized( $data, $strict = true )
absint
/**
* Convert a value to non-negative integer.
*
* @since 2.5.0
*
* @param mixed $maybeint Data you wish to have converted to a non-negative integer.
* @return int A non-negative integer.
*/
(int) function absint( $maybeint )
number_format_i18n
数字をローカライズすることができます。例えば日本であれば、
echo number_format_i18n( 1234 ); // 1,234
のように","をつけることができます。
/**
* Convert float number to format based on the locale.
*
* @since 2.3.0
*
* @global WP_Locale $wp_locale
*
* @param float $number The number to convert based on locale.
* @param int $decimals Optional. Precision of the number of decimal places. Default 0.
* @return string Converted number in string format.
*/
(string) function number_format_i18n( $number, $decimals = 0 )
wp_get_referer
リファラーを取得します。
/**
* Retrieve referer from '_wp_http_referer' or HTTP referer.
*
* If it's the same as the current request URL, will return false.
*
* @since 2.0.4
*
* @return false|string False on failure. Referer URL on success.
*/
(false|string) function wp_get_referer()
get_allowed_mime_types
ユーザーに許可されている MINE タイプとファイルの拡張子一覧を取得します。
/**
* Retrieve list of allowed mime types and file extensions.
*
* @since 2.8.6
*
* @param int|WP_User $user Optional. User to check. Defaults to current user.
* @return array Array of mime types keyed by the file extension regex corresponding
* to those types.
*/
(array) function get_allowed_mime_types( $user = null )
get_num_queries
WP 実行中のデータベースへのクエリ回数を取得します。
/**
* Retrieve the number of database queries during the WordPress execution.
*
* @since 2.0.0
*
* @global wpdb $wpdb WordPress database abstraction object.
*
* @return int Number of database queries.
*/
(int) function get_num_queries()
HTTP リクエスト関連
wp_get_http_headers
URL で指定されるページの HTTP ヘッダーを取得します。
/**
* Retrieve HTTP Headers from URL.
*
* @since 1.5.1
*
* @param string $url URL to retrieve HTTP headers from.
* @param bool $deprecated Not Used.
* @return bool|string False on failure, headers on success.
*/
(bool|string) function wp_get_http_headers( $url, $deprecated = false )
wp_remote_fopen
wp_safe_remote_get
関数を呼び出して URI のコンテンツを取得します。
/**
* HTTP request for URI to retrieve content.
*
* @since 1.5.1
*
* @see wp_safe_remote_get()
*
* @param string $uri URI/URL of web page to retrieve.
* @return false|string HTTP content. False on failure.
*/
(false|string) function wp_remote_fopen( $uri )
ファイル・ディレクトリ関連
wp_get_upload_dir
アップロードディレクトリの path と URL を配列で返します。wp_upload_dir
ではディレクトリを作ろうとするため、こちらの方が関数としては軽量です。
/**
* Retrieves uploads directory information.
*
* Same as wp_upload_dir() but "light weight" as it doesn't attempt to create the uploads directory.
* Intended for use in themes, when only 'basedir' and 'baseurl' are needed, generally in all cases
* when not uploading files.
*
* @since 4.5.0
*
* @see wp_upload_dir()
*
* @return array See wp_upload_dir() for description.
*/
(array) function wp_get_upload_dir()
wp_upload_dir
返り値は wp_get_upload_dir
と同じですが、ディレクトリがなければ作成します。
/**
* Get an array containing the current upload directory's path and url.
*
* Checks the 'upload_path' option, which should be from the web root folder,
* and if it isn't empty it will be used. If it is empty, then the path will be
* 'WP_CONTENT_DIR/uploads'. If the 'UPLOADS' constant is defined, then it will
* override the 'upload_path' option and 'WP_CONTENT_DIR/uploads' path.
*
* The upload URL path is set either by the 'upload_url_path' option or by using
* the 'WP_CONTENT_URL' constant and appending '/uploads' to the path.
*
* If the 'uploads_use_yearmonth_folders' is set to true (checkbox if checked in
* the administration settings panel), then the time will be used. The format
* will be year first and then month.
*
* If the path couldn't be created, then an error will be returned with the key
* 'error' containing the error message. The error suggests that the parent
* directory is not writable by the server.
*
* On success, the returned array will have many indices:
* 'path' - base directory and sub directory or full path to upload directory.
* 'url' - base url and sub directory or absolute URL to upload directory.
* 'subdir' - sub directory if uploads use year/month folders option is on.
* 'basedir' - path without subdir.
* 'baseurl' - URL path without subdir.
* 'error' - false or error message.
*
* @since 2.0.0
* @uses _wp_upload_dir()
*
* @staticvar array $cache
* @staticvar array $tested_paths
*
* @param string $time Optional. Time formatted in 'yyyy/mm'. Default null.
* @param bool $create_dir Optional. Whether to check and create the uploads directory.
* Default true for backward compatibility.
* @param bool $refresh_cache Optional. Whether to refresh the cache. Default false.
* @return array See above for description.
*/
(array) function wp_upload_dir( $time = null, $create_dir = true, $refresh_cache = false )
get_temp_dir
一時ファイルのための書き込み可能なディレクトリを取得します。macOS の場合は、/var/folders/...
が返ってきます。
/**
* Determine a writable directory for temporary files.
*
* Function's preference is the return value of sys_get_temp_dir(),
* followed by your PHP temporary upload directory, followed by WP_CONTENT_DIR,
* before finally defaulting to /tmp/
*
* In the event that this function does not find a writable location,
* It may be overridden by the WP_TEMP_DIR constant in your wp-config.php file.
*
* @since 2.5.0
*
* @staticvar string $temp
*
* @return string Writable temporary directory.
*/
(string) function get_temp_dir()
フォーム関連
wp_nonce_url
URL クエリに nonce を追加して返します。デフォルトでは ?_wpnonce=d6d39157de
のようなクエリが追加されます。
/**
* Retrieve URL with nonce added to URL query.
*
* @since 2.0.4
*
* @param string $actionurl URL to add nonce action.
* @param int|string $action Optional. Nonce action name. Default -1.
* @param string $name Optional. Nonce name. Default '_wpnonce'.
* @return string Escaped URL with nonce action added.
*/
(string) function wp_nonce_url( $actionurl, $action = -1, $name = '_wpnonce' )
wp_referer_field
フォームにリファラーを hidden フィールドとして追加するための input 要素を出力します。
/**
* Retrieve or display referer hidden field for forms.
*
* The referer link is the current Request URI from the server super global. The
* input name is '_wp_http_referer', in case you wanted to check manually.
*
* @since 2.0.4
*
* @param bool $echo Optional. Whether to echo or return the referer field. Default true.
* @return string Referer field HTML markup.
*/
(string) function wp_referer_field( $echo = true )